Object Oriented Programming
Offers a sustainable way to write spaghetti code and lets you accrete programs as a series of patches
What is OOP?
What the four basic OOP Programming?
1. Abstraction.
Abstraction means using simple things to represent complexity.
Example: A car in itself is a well-defined object, which is composed of several other smaller objects like a gearing system, steering mechanism, engine, which are again have their own subsystems. But for humans car is a one single object, which can be managed by the help of its subsystems, even if their inner details are unknown.
2. Encapsulation.
This is the practice of keeping fields within a class private, then providing access to them via public methods.
An example of encapsulation is the class of java.util.Hashtable. User only knows that he can store data in the form of key/value pair in a Hashtable and that he can retrieve that data in the various ways. But the actual implementation like, how and where this data is actually stored, is hidden from the user. User can simply use Hashtable wherever he wants to store Key/Value pairs without bothering about its implementation.
3. Inheritance.
It lets programmers create new classes that share some of the attributes of existing classes. This lets us build on previous work without reinventing the wheel. For example, you could create the class Car that specifies wheels = 4 and a subclass Sedan that includes the attribute doors = 4. The flow of inheritance relationships often reflects the logical relationship similar to squares and rectangles; in this case, all sedans are cars, but not all cars are sedans.
4. Polymorphism.
This concept lets programmers use the same word to mean different things in different contexts. For example, the human body has different organs. Every organ has a different function to perform; the heart is responsible for blood flow, lungs for breathing, brain for cognitive activity and kidneys for excretion. So we have a standard method function that performs differently depending upon the organ of the body.
Object-Oriented Requirements Models
The models requires in OOP are class model, state model, and interaction model. The class model shows all the classes inside the system. The state model, defines the actions inside of a state diagram. The interaction model is used to show various interactions between objects, one of example of this is Sequence Diagram. In object-oriented requirements analysis, you should model real-world entities using object classes. You may create different types of object model, showing how object classes are related to each other, how objects are aggregated to form other objects, how objects interact with other objects and so on.
Tasks
You need to design a Vending Machine which
- Accepts coins of 1,5,10,25 Cents i.e. penny, nickel, dime, and quarter.
- Allow user to select products Coke(25), Pepsi(35), Soda(45)
- Allow user to take refund by canceling the request.
- Return the selected product and remaining change if any
- Allow reset operation for vending machine supplier.
Class Name | Description |
---|---|
VendingMachine | It defines the public API of a vending machine, usually, all high-level functionality should go in this class |
VendingMachineImpl | A sample implementation of Vending Machine |
VendingMachineFactory | A Factory class to create different kinds of Vending Machine |
Item | Java Enum to represent Item served by Vending Machine |
Inventory | Java class to represent an Inventory, used for creating the case and item inventory inside Vending Machine |
Coin | Another Java Enum to represent Coins supported by Vending Machine |
Bucket | A parameterized class to hold two objects. It’s kind of Pair class. |
NotFullPaidException | An Exception is thrown by Vending Machine when a user tries to collect an item, without paying the full amount. |
NotSufficientChangeException | Vending Machine throws this exception to indicate that it doesn’t have sufficient change to complete this request. |
SoldOutException | Vending Machine throws this exception if the user requests a product that is sold out. |
B. SONGSALES
Imagine that you and your band have just recorded a song, and you want to permit fans to purchase and download the song over the Internet. Your manager receives a commission equal to 12% of the total sales revenue for your song. and the Internet site that list your song receives a 3% commission.
Design a Java Program that ask for the title of the song, the purchase price of the song (in peso and cents), and the number of copies of the song that have been sold. Calculate the total revenue generated by your song, the commission received by your manager, and the commission received by the Internet site.
C. SONGSALES_GUI
Redesign the program on SongSales, which you created in Task C. Use a dialog box to ask the user for the title of the song, the purchase price of the song (in peso and cents), and the number of copies of the song that have been sold. Calculate the total revenue generated by your song, the commission received by your manager, and the commission received by the Internet site.
D. LEAP YEAR OR NOT?
Write a program that replies either Leap Year or Not a Leap Year, given a year. It is a leap year if the year is divisible by 4 but not by 100 (for example, 1796 is a leap year because it is divisible by 4 but not by 100). A year that is divisible by both 4 and 100 is a leap year if it is also divisible by 400 (for example, 2000 is a leap year, but 1800 is not).
E. EMPLOYEES AT MYJAVA LO-FAT BURGER
Employees at MyJava Lo-Fat Burgers earn the basic hourly wage of $7.25. They will receive time-and-a-half of their basic rate for overtime hours. In addition, they will receive a commission on the sales they generate while tending the counter. The commission is based on the following formula
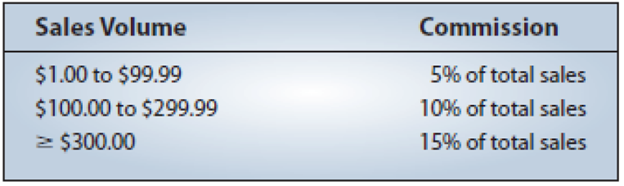